PHP Question and Answer.
PHP Question bang
1.What is PHP? List of feature of php?
Ans :-
PHP (Hypertext Preprocessor) is a server-side scripting language that is widely used for web development. It was originally designed for creating dynamic web pages, but it has since evolved to become a versatile language that can be used for a wide range of applications. Here are some of the features of PHP:
Easy to learn: PHP has a relatively simple syntax that is easy to learn and understand, even for beginners.
Cross-platform compatibility: PHP can run on a wide range of platforms, including Windows, Linux, macOS, and many others.
Open source: PHP is an open-source language, which means that it is freely available and can be modified and distributed by anyone.
Large developer community: PHP has a large and active developer community, which means that there is a lot of support and resources available for those who are learning the language.
Powerful string handling capabilities: PHP has powerful built-in functions for manipulating strings, which makes it particularly useful for working with text-based data.
Server-side scripting: PHP is primarily used for server-side scripting, which means that it can be used to generate dynamic content and interact with databases.
Object-oriented programming support: PHP supports object-oriented programming (OOP), which allows developers to create more modular and reusable code.
Scalable: PHP can be used to build large-scale applications that can handle a high volume of traffic and data.
Compatibility with other technologies: PHP can be used in conjunction with other technologies, such as HTML, CSS, and JavaScript, to create dynamic web pages and applications.
Security: PHP has built-in security features, such as the ability to prevent SQL injection attacks and cross-site scripting (XSS) attacks.
2. How to place single line and multiline comment in php
Ans :-
In PHP, you can use both single-line and multi-line comments to document your code and make it more readable.To add a single-line comment, you can use two forward slashes (//) followed by your comment text.Anything after the double forward slashes will be ignored by the PHP interpreter.
To add a multi-line comment, you can use a forward slash followed by an asterisk to start the comment block, and then use an asterisk followed by a forward slash to end the comment block.Anything between the /* and */ symbols will be ignored by the PHP interpreter, even if it spans multiple lines.It’s good practice to add comments to your code to make it more understandable and easier to maintain.
3.List out data types in php.
Ans :-
PHP supports various data types that can be used to store different kinds of data. Here are the data types supported by PHP:
Integer: Integers are whole numbers without any decimal point. They can be either positive, negative or zero.
Float: Floats (also called doubles) are numbers with decimal points or scientific notation.
String: Strings are sequences of characters enclosed in single or double quotes.
Boolean: Booleans are values that can be either true or false.
Array: Arrays are ordered collections of values, which can be of different data types.
Object: Objects are instances of classes, which can have properties and methods.
Null: Null is a special data type that represents the absence of a value.
Resource: Resources are special variables that hold references to external resources such as database connections or file handles.
Callable: Callable is a pseudo-type that represents a function or method that can be called as a callback.
Mixed: Mixed is a data type that can represent any data type.
Void: Void is a special data type used for functions that do not return a value.
It’s important to understand data types in PHP because they affect how data is stored and processed, and can have an impact on the performance and behavior of your code.
4.What is the scope of super-global variable in php.
Ans :-
In PHP, super-global variables are accessible from any part of the script, regardless of their scope. They are called “super-global” because they are predefined variables that can be accessed from anywhere in the script, including inside functions and methods, without the need for any special syntax or declaration.
Here are the most commonly used super-global variables in PHP:
$GLOBALS: This variable is an array that contains all global variables in the current script, including those that are defined outside of functions.
$_SERVER: This variable is an array that contains information about the server and the execution environment, such as the request method, server name, and script name.
$_GET: This variable is an array that contains the values of the query string parameters that were passed in the URL.
$_POST: This variable is an array that contains the values of the form fields that were submitted using the HTTP POST method.
$_FILES: This variable is an array that contains information about uploaded files, such as the file name, size, and type.
$_COOKIE: This variable is an array that contains the values of cookies that were set in the current session.
$_SESSION: This variable is an array that contains the values of session variables that were set in the current session.
$_REQUEST: This variable is an array that contains the values of $_GET, $_POST, and $_COOKIE combined.
It’s important to be careful when using super-global variables, as they can be easily modified and can introduce security vulnerabilities in your code. You should always validate and sanitize user input before using it in your code, especially when using super-global variables like $_GET and $_POST.
5.Justify answer Php is loosely type language.
Ans :-
PHP is a loosely typed language because it allows variables to be assigned values of different types without explicitly declaring the data type. In other words, variables in PHP are not bound to a specific data type and can hold values of different data types at different times.
For example, a variable in PHP can hold an integer value, then be assigned a string value, and later hold a boolean value. PHP will automatically convert the value to the appropriate data type as needed.
This is different from strongly typed languages like Java or C++, where variables must be explicitly declared with a specific data type and cannot be assigned values of other data types.
The loose typing in PHP can make it easier to write and maintain code, as it allows for greater flexibility and reduces the need for type checking and type casting. However, it also means that variables can be accidentally assigned unexpected values, which can lead to bugs and errors in the code. It’s important to use good programming practices and validate input data to prevent these issues.
6.What is die(), exit(),return() in php.
Ans :-
In PHP, die(), exit(), and return() are language constructs used to control the flow of execution and terminate a script or function under certain conditions.
die() and exit() are essentially the same thing and can be used interchangeably. They immediately terminate the script execution and output a message to the browser.
For example:
if ($error) {
die(“An error occurred: $error”);
}
In this example, if the $error variable is true, the script will terminate and display the error message to the user.
return(), on the other hand, is used within functions to exit the function and return a value to the calling code.
For example:
function addNumbers($a, $b) {
$sum = $a + $b;
return $sum;
}
In this example, the addNumbers() function takes two parameters and returns their sum. The return statement is used to exit the function and return the value of $sum to the calling code.
It’s important to use these constructs carefully and only in appropriate situations, as prematurely terminating the script or function can cause unexpected behavior and errors
7.Write syntax of foreach loop in php
Ans :- The foreach loop in PHP is used to iterate over an array or an object, and execute a block of code for each element in the array or object. Here is the syntax for the foreach loop in PHP:
foreach ($array as $value) {
// code to be executed
}
In this syntax, $array is the array or object that you want to iterate over, and $value is a variable that represents the current element of the array or object during each iteration.
You can also include the key of each element in the loop by using the following syntax:
foreach ($array as $key => $value) {
// code to be executed
}
In this syntax, $key represents the key of the current element, and $value represents the value of the current element.
Here’s an example of how you can use the foreach loop to iterate over an array:
$fruits = array(“apple”, “banana”, “orange”);
foreach ($fruits as $fruit) {
echo $fruit . “<br>”;
}
In this example, the foreach loop iterates over the $fruits array and prints each fruit name to the screen.
Note that you can use any variable name instead of $value or $key, as long as it is a valid variable name in PHP.
8.Write syntax of exit control loop in php
Ans :-
In PHP, you can use an exit control loop to prematurely terminate a loop based on a certain condition. Here’s the syntax for the exit control loop in PHP:while
(condition) {
// code to be executed
if (exit condition) {
break;
}
}
In this syntax, the while loop executes the code block as long as the condition is true. Within the loop, you can check for an exit condition using an if statement. If the exit condition is met, you can use the break statement to terminate the loop and exit the loop block.
Here’s an example of how you can use the exit control loop to terminate a loop based on a certain condition:
$numbers = array(1, 2, 3, 4, 5);
foreach ($numbers as $number) {
if ($number == 3) {
break;
}
echo $number . “<br>”;
}
In this example, the foreach loop iterates over the $numbers array and prints each number to the screen. However, if the current number is equal to 3, the break statement is executed and the loop is terminated.
Note that you can use the exit control loop with any type of loop in PHP, including while, do-while, and for loops.
9.Write syntax of entry control loop in php
Ans :-
In PHP, you can use an entry control loop to execute a loop based on a certain condition. Here’s the syntax for the entry control loop in PHP:
while (condition) {
// code to be executed
}
In this syntax, the while loop executes the code block as long as the condition is true. The loop block is entered only if the condition is true from the start.
Here’s an example of how you can use the while loop to execute a loop based on a certain condition:
$counter = 1;
while ($counter <= 5) {
echo $counter . “<br>”;
$counter++;
}
In this example, the while loop iterates over the $counter variable and prints each number to the screen as long as the $counter value is less than or equal to 5.
Note that you can use the entry control loop with any type of loop in PHP, including while, do-while, and for loops. However, the syntax for each type of loop is slightly different.
10.Differentiate between break and continue statement in php.
Ans :- In PHP, both break and continue are used within loops to control the flow of execution. However, their effects are different.
break: When the break statement is encountered within a loop, the loop is immediately terminated and control is transferred to the first statement after the loop. This means that the loop block will not be executed any further.
Here’s an example of how the break statement works in PHP:
for ($i = 1; $i <= 5; $i++) {
if ($i == 3) {
break;
}
echo $i . “<br>”;
}
In this example, the for loop iterates over the values of $i from 1 to 5. However, when the $i value is 3, the break statement is executed and the loop is terminated. As a result, only the numbers 1 and 2 are printed to the screen.
continue: When the continue statement is encountered within a loop, the current iteration of the loop is immediately terminated and control is transferred to the next iteration of the loop. This means that the loop block will continue to execute, but the current iteration will be skipped.
Here’s an example of how the continue statement works in PHP:
for ($i = 1; $i <= 5; $i++) {
if ($i == 3) {
continue;
}
echo $i . “<br>”;
}
In this example, the for loop iterates over the values of $i from 1 to 5. However, when the $i value is 3, the continue statement is executed and the current iteration of the loop is terminated. As a result, the number 3 is skipped, and the numbers 1, 2, 4, and 5 are printed to the screen.
In summary, break is used to terminate a loop prematurely, while continue is used to skip the current iteration of the loop and move on to the next iteration.
OR
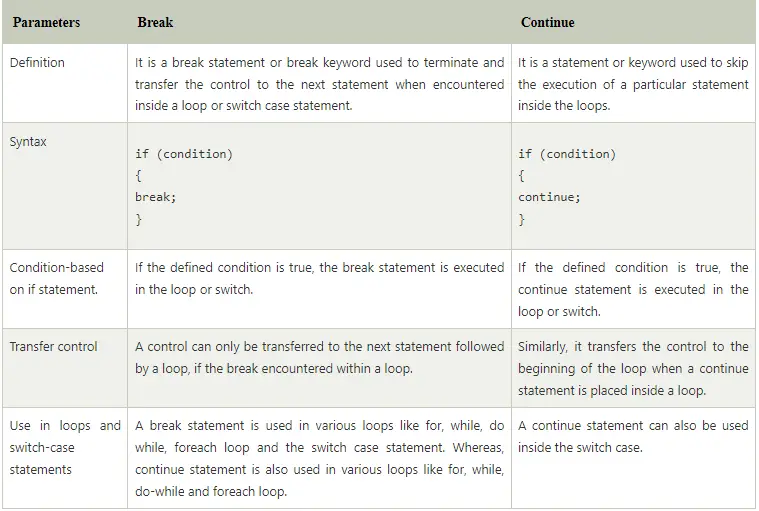
11.Write syntax to create switch case in php.
Ans :-
In PHP, the switch statement is used to perform different actions based on different conditions. Here’s the basic syntax for the switch statement:
switch (variable) {
case value1:
// code to be executed if variable equals value1
break;
case value2:
// code to be executed if variable equals value2
break;
// more cases can be added here
default:
// code to be executed if none of the above cases are true
break;
}
In this syntax, the switch statement evaluates the variable and compares it to the different case values. If the variable matches a case value, the corresponding code block is executed. If none of the case values match the variable, the default code block is executed.
Important points to be noticed about switch case:
1.The default is an optional statement. Even it is not important, that default must always be the last statement.
2.There can be only one default in a switch statement. More than one default may lead to a Fatal error.
3.Each case can have a break statement, which is used to terminate the sequence of statement.
4.The break statement is optional to use in switch. If break is not used, all the statements will execute after finding matched case value.
5.PHP allows you to use number, character, string, as well as functions in switch expression.
6.Nesting of switch statements is allowed, but it makes the program more complex and less readable.
7.You can use semicolon (;) instead of colon (:). It will not generate any error.
Program to check Vowel and consonant
We will pass a character in switch expression to check whether it is vowel or constant. If the passed character is A, E, I, O, or U, it will be vowel otherwise consonant.
<?php
$ch = ‘U’;
switch ($ch)
{
case ‘a’:
echo “Given character is vowel”;
break;
case ‘e’:
echo “Given character is vowel”;
break;
case ‘i’:
echo “Given character is vowel”;
break;
case ‘o’:
echo “Given character is vowel”;
break;
case ‘u’:
echo “Given character is vowel”;
break;
case ‘A’:
echo “Given character is vowel”;
break;
case ‘E’:
echo “Given character is vowel”;
break;
case ‘I’:
echo “Given character is vowel”;
break;
case ‘O’:
echo “Given character is vowel”;
break;
case ‘U’:
echo “Given character is vowel”;
break;
default:
echo “Given character is consonant”;
break;
}
?>
12. List out sorting function of array in php.
Ans :- PHP provides various built-in functions to sort arrays in different ways. Here’s a list of some common sorting functions in PHP:
sort() – Sorts an array in ascending order based on the value. The keys are not preserved.
Example :-
<?php
$cars = array(“Volvo”, “BMW”, “Toyota”);
sort($cars);
?>
rsort() – Sorts an array in descending order based on the value. The keys are not preserved.
Example :-
<?php
$cars = array(“Volvo”, “BMW”, “Toyota”);
rsort($cars);
?>
asort() – Sorts an array in ascending order based on the value. The keys are preserved.
Example :-
<?php
$age = array(“Peter”=>”35”, “Ben”=>”37”, “Joe”=>”43”);
asort($age);
?>
arsort() – Sorts an array in descending order based on the value. The keys are preserved.
Example :-
<?php
$age = array(“Peter”=>”35”, “Ben”=>”37”, “Joe”=>”43”);
arsort($age);
?>
ksort() – Sorts an array in ascending order based on the key. The values are preserved.
Example :-
<?php
$age = array(“Peter”=>”35”, “Ben”=>”37”, “Joe”=>”43”);
ksort($age);
?>
krsort() – Sorts an array in descending order based on the key. The values are preserved.
Example :-
<?php
$age = array(“Peter”=>”35”, “Ben”=>”37”, “Joe”=>”43”);
krsort($age);
?>
13.List out any five array function in php except sorting function.
Ans :-
Sure, here are five common array functions in PHP that are not related to sorting:
array_push() – Adds one or more elements to the end of an array.
array_pop() – Removes the last element from an array.
array_slice() – Returns a slice of an array based on a specified start and end index.
array_merge() – Merges two or more arrays into a single array.
array_search() – Searches an array for a given value and returns the corresponding key if found.
1. array_push() – Adds one or more elements to the end of an array.
Syntax:
array_push(array $array, mixed …$values): int
Example:
$fruits = array(“apple”, “banana”);
array_push($fruits, “orange”, “pear”);
print_r($fruits);
Output:
Array
(
[0] => apple
[1] => banana
[2] => orange
[3] => pear
)
2. array_pop() – Removes the last element from an array.
Syntax:
array_pop(array &$array): mixed
Example:
$fruits = array(“apple”, “banana”, “orange”, “pear”);
$last_fruit = array_pop($fruits);
echo $last_fruit;
Output:
pear
3. array_shift() – Removes the first element from an array.
Syntax:
array_shift(array &$array): mixed
Example:
$fruits = array(“apple”, “banana”, “orange”, “pear”);
$first_fruit = array_shift($fruits);
echo $first_fruit;
Output:
apple
4.array_slice() – Extracts a slice of an array.
Syntax:
array_slice(array $array, int $offset, ?int $length = null, bool $preserve_keys = false): array
Example:
$fruits = array(“apple”, “banana”, “orange”, “pear”);
$slice = array_slice($fruits, 1, 2);
print_r($slice);
Output:
Array
(
[0] => banana
[1] => orange
)
5. array_merge() – Merges one or more arrays into a single array.
Syntax:
array_merge(array …$arrays): array
Example:
$fruits1 = array(“apple”, “banana”);
$fruits2 = array(“orange”, “pear”);
$fruits = array_merge($fruits1, $fruits2);
print_r($fruits);
Output:
Array
(
[0] => apple
[1] => banana
[2] => orange
[3] => pear
)
14. List out different types of validation required of php form
Ans :-
There is no guarantee that the information provided by the user is always correct. PHP validates the data at the server-side, which is submitted by HTML form. You need to validate a few things:
Empty String
Validate String
Validate Numbers
Validate Email
Validate URL
Input length
1. Required Field Validation: This ensures that a form field is not empty or null.
Syntax:
if(empty($_POST[‘field_name’])) {
$errors[] = ‘Field is required’;
}
Example :-
<form method=”post” action=””>
<label for=”name”>Name:</label>
<input type=”text” name=”name” id=”name”>
<button type=”submit”>Submit</button>
</form>
<?php
if($_SERVER[‘REQUEST_METHOD’] == ‘POST’) {
if(empty($_POST[‘name’])) {
$errors[] = ‘Name is required’;
}
}
?>
2. Email Validation: This ensures that an email field is a valid email format.
Syntax:
if(!filter_var($_POST[’email’], FILTER_VALIDATE_EMAIL)) {
$errors[] = ‘Invalid email format’;
}
Example:
<form method=”post” action=””>
<label for=”email”>Email:</label>
<input type=”email” name=”email” id=”email”>
<button type=”submit”>Submit</button>
</form>
<?php
if($_SERVER[‘REQUEST_METHOD’] == ‘POST’) {
if(!filter_var($_POST[’email’], FILTER_VALIDATE_EMAIL)) {
$errors[] = ‘Invalid email format’;
}
}
?>
3. Numeric Validation: This ensures that a field contains only numeric values.
Syntax
if(!is_numeric($_POST[‘age’])) {
$errors[] = ‘Age must be numeric’;
}
Example :-
<form method=”post” action=””>
<label for=”age”>Age:</label>
<input type=”text” name=”age” id=”age”>
<button type=”submit”>Submit</button>
</form>
<?php
if($_SERVER[‘REQUEST_METHOD’] == ‘POST’) {
if(!is_numeric($_POST[‘age’])) {
$errors[] = ‘Age must be numeric’;
}
}
?>
4.Password Validation: This ensures that a password field meets certain criteria, such as minimum length and use of special characters.
Syntax:
if(strlen($_POST[‘password’]) < 8) {
$errors[] = ‘Password must be at least 8 characters long’;
}
<form method=”post” action=””>
<label for=”password”>Password:</label>
<input type=”password” name=”password” id=”password”>
<button type=”submit”>Submit</button>
</form>
<?php
if($_SERVER[‘REQUEST_METHOD’] == ‘POST’) {
if(strlen($_POST[‘password’]) < 8) {
$errors[] = ‘Password must be at least 8 characters long’;
}
}
?>
5.File Upload Validation: This ensures that a file upload meets certain criteria, such as file type and file size.
Syntax:
if($_FILES[‘file’][‘type’] != ‘image/jpeg’ || $_FILES[‘file’][‘size’] > 500000) {
$errors[] = ‘Invalid file format or file size too large’;
}
Example:
<form method=”post” action=”” enctype=”multipart/form-data”>
<label for=”file”>File:</label>
<input type=”file” name=”file” id=”file”>
<button type=”submit”>Submit</button>
</form>
<?php
if($_SERVER[‘REQUEST_METHOD’] == ‘POST’) {
if($_FILES[‘file’][‘type’] != ‘image/jpeg’ || $_FILES[‘file’][‘size’]
15.Which are super-global variable is used to passing form data.
Ans :-The super-global variable used to pass form data in PHP is $_POST. It is an associative array that contains key-value pairs of form data that is submitted using the HTTP POST method. The keys in the $_POST array correspond to the name attribute of the form input fields, and the values are the user-submitted data.
For example, if you have a form with an input field like this:
<html>
<body>
<form method=”post” action=”<?php echo $_SERVER[‘PHP_SELF’];?>”>
Name: <input type=”text” name=”fname”>
<input type=”submit”>
</form>
<?php
if ($_SERVER[“REQUEST_METHOD”] == “POST”) {
// collect value of input field
$name = $_POST[‘fname’];
if (empty($name)) {
echo “Name is empty”;
} else {
echo $name;
}
}
?>
</body>
</html>
You can use the $_POST super-global to access and validate form data submitted by the user, and use it to perform various actions like storing the data in a database or sending it via email. It is important to properly sanitize and validate user input to prevent security vulnerabilities in your PHP application.
16.List out any five file handling function in php.
Ans :-
File handling in PHP refers to the process of working with files on the server, such as reading from or writing to files, creating, deleting or modifying files, and more.
Here are five commonly used file handling functions in PHP:
1. fopen()
2.fclose()
3.fgets()
4.fwrite()
5.file_exists()
1. fopen(): This function is used to open a file and returns a file pointer resource. It takes two parameters: the file name (including the path) and the mode in which to open the file.
Syntax:
resource fopen ( string $filename , string $mode [, bool $use_include_path = false [, resource $context ]] )
or
$file_handle = fopen($file_name, $mode);
Example :-
<?php
$handle = fopen(“c:\\folder\\file.txt”, “r”);
?>
2. fclose(): This function is used to close an open file pointer. It takes a single parameter, the file pointer resource.
Syntax:
fclose($file_handle);
Example :-
<?php
fclose($handle);
?>
3. fgets(): This function is used to read a single line from a file. It takes a single parameter, the file pointer resource.
Syntax:
$line = fgets($file_handle);
Example
$line = fgets($file_handle);
4.fwrite(): This function is used to write data to a file. It takes two parameters: the file pointer resource and the data to be written.
Syntax:
fwrite($file_handle, $data);
Example:-
fwrite($file_handle, “Hello World!”);
5. file_exists(): This function is used to check if a file exists. It takes a single parameter, the file name (including the path).
Syntax:
if (file_exists($file_name)) {
// file exists
} else {
// file does not exist
}
Example
<?php
$filename = ‘readme.txt’;
if (file_exists($filename)) {
$message = “The file $filename exists”;
} else {
$message = “The file $filename does not exist”;
}
echo $message;
17. List out any six string function in php.
Ans :- In PHP, string functions are built-in functions that allow you to manipulate and work with strings. These functions can perform a wide range of operations on strings such as finding the length of a string, converting strings to uppercase or lowercase, searching for a specific character or substring within a string, and many more.
Some of the most commonly used string functions in PHP include strlen(), str_replace(), substr(), strtoupper(), strtolower(), and strpos().
1. strlen() – returns the length of a string in PHP.
Syntax: strlen(string $string): int
Example:
$string = “Hello, World!”;
$length = strlen($string);
echo “The length of the string is: ” . $length;
// Output: The length of the string is: 13
2.str_replace() – searches and replaces all occurrences of a string with another string.
Syntax: str_replace(mixed $search, mixed $replace, mixed $subject, int &$count): mixed
Example:
$string = “The quick brown fox jumps over the lazy dog.”;
$new_string = str_replace(“fox”, “cat”, $string);
echo $new_string;
// Output: The quick brown cat jumps over the lazy dog.
3.substr() – returns a portion of a string starting from a given position and for a specified length.
Syntax: substr(string $string, int $start, ?int $length = null): string
Example:
$string = “Hello, World!”;
$substring = substr($string, 0, 5);
echo $substring;
// Output: Hello
4. strtoupper() – converts a string to uppercase.
Syntax: strtoupper(string $string): string
Example:
$string = “Hello, World!”;
$uppercase_string = strtoupper($string);
echo $uppercase_string;
// Output: HELLO, WORLD!
5.strtolower() – converts a string to lowercase.
Syntax: strtolower(string $string): string
Example:
$string = “Hello, World!”;
$lowercase_string = strtolower($string);
echo $lowercase_string;
// Output: hello, world!
6.strpos() – searches for a string inside another string and returns the position of the first occurrence.
Syntax: strpos(string $haystack, mixed $needle, int $offset = 0): int|false
Example:
$string = “The quick brown fox jumps over the lazy dog.”;
$position = strpos($string, “fox”);
echo $position;
// Output: 16
18.List out any five math function in php.
Ans :- In PHP, math functions are built-in functions that allow you to perform mathematical operations on numbers. These functions can be used to perform basic arithmetic operations like addition, subtraction, multiplication, and division, as well as more advanced operations like exponentiation, logarithms, trigonometric functions, and rounding.
five commonly used math functions in PHP:
1. abs() – returns the absolute (positive) value of a number.
Syntax: abs(mixed $number): float|int
Example:
$number = -10;
$absolute_value = abs($number);
echo $absolute_value;
// Output: 10
2.pow() – returns the result of raising a number to a given power.
Syntax: pow(float $base, float $exponent): float
Example:
$base = 2;
$exponent = 3;
$result = pow($base, $exponent);
echo $result;
// Output: 8
3.sqrt() – returns the square root of a number.
Syntax: sqrt(float $number): float
Example:
$number = 25;
$square_root = sqrt($number);
echo $square_root;
// Output: 5
4.rand() – generates a random integer between two given numbers.
Syntax: rand(int $min, int $max): int
Example:
$random_number = rand(1, 10);
echo $random_number;
// Output: a random number between 1 and 10
5.ceil() – rounds a number up to the nearest integer.
Syntax: ceil(float $number): float
Example:
$number = 3.14;
$rounded_up = ceil($number);
echo $rounded_up;
// Output: 4
19. Different between echo and print in php.
Ans :- In PHP, both echo and print are used to output text or variables to the browser or to a file. However, there are some differences between the two:
1. Syntax: echo is a language construct and doesn’t require parentheses, while print is a function and requires parentheses.
echo “Hello, world!”;
print(“Hello, world!”);
2.Return value: echo doesn’t return any value, while print returns 1 if it successfully outputs the string.
$result = echo “Hello, world!”; // Error
$result = print(“Hello, world!”); // Outputs “Hello, world!” and sets $result to 1
3.Performance: echo is faster than print since it doesn’t return a value and doesn’t require parentheses.
In most cases, the difference in performance is negligible, so the choice between echo and print usually comes down to personal preference and coding style.
4.Multiple parameters: echo can accept multiple parameters separated by commas, while print can only accept one parameter.
$name = “John”;
$age = 30;
echo “My name is “, $name, ” and I am “, $age, ” years old.”;
// Output: My name is John and I am 30 years old.
print “My name is $name and I am $age years old.”;
// Output: My name is John and I am 30 years old.
20.Write syntax to create used defined function in php.
Ans :- To create a user-defined function in PHP, you can use the function keyword followed by the name of the function, any parameters it accepts, and the code that it should execute. Here is the basic syntax for creating a user-defined function:
function function_name($parameter1, $parameter2, …) {
// code to be executed
}
Here’s an example of a user-defined function that takes two parameters and returns the sum of the two numbers:
function sum($a, $b) {
$result = $a + $b;
return $result;
}
In the example above, the function is named sum, and it accepts two parameters, $a and $b. The function calculates the sum of the two numbers and assigns it to the variable $result. Finally, the function returns the value of $result.
You can call this function like this:
$result = sum(2, 3);
echo $result; // Output: 5
21.Write syntax to create session in php.
Ans :- A session in PHP is a way to store and access data across multiple pages or requests from the same user. Sessions work by storing data on the server and associating it with a unique session ID, which is then sent to the client’s browser as a cookie or URL parameter. This allows the server to identify the user and retrieve the stored data each time the user makes a request.
Here is the basic syntax for starting a session in PHP:
<?php
session_start();
?>
This code should be placed at the top of each page that needs to access the session data. Once a session has been started, you can store and retrieve data using the $_SESSION superglobal variable. Here’s an example:
<?php
session_start();
// Set session variables
$_SESSION[‘name’] = ‘John’;
$_SESSION[‘age’] = 30;
// Retrieve session variables
$name = $_SESSION[‘name’];
$age = $_SESSION[‘age’];
// Output session variables
echo “Name: $name, Age: $age”;
?>
In the example above, we start the session using session_start(). We then set two session variables using the $_SESSION superglobal, name and age, and assign them values. We then retrieve these variables and assign them to local variables $name and $age, respectively. Finally, we output the values of these variables using echo.
It’s important to note that sessions should be used with caution, as they can consume server resources and may not work as expected in certain scenarios, such as when using a load balancer or caching. Additionally, storing sensitive data in sessions can pose a security risk if the session data is not properly secured.
22. Write syntax to create cookie in php.
Ans :- A cookie in PHP is a small piece of data that is sent from a website to a user’s web browser and stored on the user’s computer. Cookies are often used to store user preferences or to track user activity across multiple pages or visits to a website. In PHP, you can create and retrieve cookies using the setcookie() and $_COOKIE functions, respectively.
Here is the basic syntax for creating a cookie in PHP using the setcookie() function:
setcookie(name, value, expire, path, domain, secure, httponly);
Here’s what each parameter in the setcookie() function represents:
name: The name of the cookie.
value: The value of the cookie.
expire: The expiration time of the cookie, in seconds since the Unix epoch. If set to 0, the cookie will expire when the user’s browser is closed. If omitted, the cookie will expire after the default time period, which is typically 30 days.
path: The path on the server where the cookie will be available. If set to ‘/’, the cookie will be available across the entire domain.
domain: The domain that the cookie is available to. If set to the domain of the current website, the cookie will be available to all pages on the site.
secure: Specifies whether the cookie should only be transmitted over a secure HTTPS connection.
httponly: Specifies whether the cookie should only be accessible via HTTP and not by JavaScript.
Here’s an example of how to create a cookie in PHP:
<?php
// Set a cookie
setcookie(‘name’, ‘John’, time() + (86400 * 30), ‘/’);
// Retrieve a cookie
$name = $_COOKIE[‘name’];
echo “Hello, $name!”;
?>
In the example above, we create a cookie called name with the value John and an expiration time of 30 days from the current time. We set the path to ‘/’, which makes the cookie available across the entire domain. We then retrieve the value of the name cookie using the $_COOKIE superglobal and output a message using echo.
It’s important to note that cookies can be a potential security risk if sensitive information is stored in them, as they are stored on the user’s computer and can be accessed by other websites or malicious actors. Therefore, it’s best to only store non-sensitive information in cookies, or to encrypt any sensitive data before storing it in a cookie.
23.Which is php function used to delete session in php.
Ans :- In PHP, the session_destroy() function is used to destroy all of the data associated with the current session. This includes all session variables and the session ID itself. Here’s the basic syntax for using session_destroy():
session_destroy();
When session_destroy() is called, it will remove all data associated with the current session, but it will not remove any cookies associated with the session. To remove the session cookie, you can use the setcookie() function with a negative expiration time, like this:
setcookie(session_name(), ”, time()-3600, ‘/’);
This code sets the value of the session cookie to an empty string and sets the expiration time to one hour in the past. The path parameter should match the path used when the cookie was originally created.
It’s important to note that calling session_destroy() does not unset any of the session variables, so they will still be available until the script completes. To completely remove all session data and unset all session variables, you should also call session_unset() before calling session_destroy():
session_unset();
session_destroy();
This will ensure that all session data is removed and that any memory used by the session is freed up.
24.Different between session_destory and session_unset in php.
Ans :- In PHP, session_destroy() and session_unset() are two different functions that are used to manage sessions.
session_destroy() is used to destroy all data associated with the current session. This includes all session variables and the session ID itself. When session_destroy() is called, all session data is removed from the server, and the client’s session cookie is removed from the client’s browser. Note that session_destroy() does not unset any of the session variables, so they will still be available until the script completes.
On the other hand, session_unset() is used to unset all session variables. This means that all data stored in session variables will be deleted, but the session itself will still be active. The session ID and any other session data will remain intact. session_unset() is often used in conjunction with session_destroy(), as it ensures that all session variables are deleted before the session is destroyed.
In summary, the main difference between session_destroy() and session_unset() is that session_destroy() destroys the entire session, including the session ID and any session data, while session_unset() only unsets the session variables, leaving the session ID and any other session data intact.
25.Write to step create and delete cookie in php.
Ans :- To create a cookie in PHP, you can use the setcookie() function. Here are the basic steps:
Step 1: Use the setcookie() function to set the cookie value. The basic syntax for the setcookie() function is:
setcookie(name, value, expire, path, domain, secure, httponly);
name: The name of the cookie.
value: The value of the cookie.
expire: The expiration time of the cookie (in seconds). If set to 0, the cookie will expire at the end of the session.
path: The path on the server where the cookie will be available.
domain: The domain where the cookie will be available.
secure: Indicates whether the cookie should only be transmitted over a secure HTTPS connection.
httponly: Indicates whether the cookie should only be accessible through the HTTP protocol.
Here’s an example that sets a cookie with the name “username” and the value “JohnDoe”:
setcookie(‘username’, ‘JohnDoe’, time() + (86400 * 30), ‘/’);
This sets the cookie to expire in 30 days and makes it available to all pages on the site.
Step 2: To delete a cookie, you can use the setcookie() function again, but with an expiration time in the past. This will cause the browser to delete the cookie. Here’s an example:
setcookie(‘username’, ”, time() – 3600, ‘/’);
This sets the expiration time of the cookie to one hour ago, which will cause the browser to delete the cookie.
Note that when deleting a cookie, you should use the same path and domain that were used when the cookie was originally set, otherwise the browser may not be able to find the cookie and delete it correctly.
26. How to create class object in php.
Ans :- In PHP, you can create a class object using the new keyword. Here’s the basic syntax for creating a class object:
$object = new ClassName();
In this syntax, ClassName is the name of the class you want to create an object of, and $object is the variable that will hold the object.
Here’s an example that creates an object of a class named Person:
class Person {
public $name;
public $age;
public function __construct($name, $age) {
$this->name = $name;
$this->age = $age;
}
public function sayHello() {
echo “Hello, my name is ” . $this->name . ” and I am ” . $this->age . ” years old.”;
}
}
$person = new Person(“John”, 30);
$person->sayHello();
In this example, we define a Person class with two public properties, $name and $age, and a constructor method that takes two arguments and initializes the properties. We also define a sayHello() method that echoes a greeting message.
Then, we create a new Person object using the new keyword and pass in the name and age arguments to the constructor. Finally, we call the sayHello() method on the object to output the greeting message.
Note that the new keyword can be used to create objects of any class, not just the Person class in this example.
27. What is method overloading and overriding.
Ans :- Method overloading and overriding are two important concepts in object-oriented programming that allow developers to create more flexible and modular code.
Method Overloading:
Method overloading is the ability to define a method with the same name as an existing method in a class, but with a different set of parameters. This allows you to create methods with the same name, but different functionality based on the parameters they receive.
In PHP, method overloading is achieved using the __call() magic method. This method is called automatically when a non-existent method is called on an object. The __call() method can be used to check the method name and parameters, and then call the appropriate method with the correct set of parameters.
Method Overriding:
Method overriding is the ability to define a method in a subclass that has the same name and signature as a method in the parent class. This allows you to replace or modify the functionality of the parent class method in the subclass.
In PHP, method overriding is achieved by simply defining a method with the same name and signature as a method in the parent class. When the method is called on an object of the subclass, the subclass method will be executed instead of the parent class method.
Here’s an example of method overloading and overriding in PHP:
class Animal {
public function speak() {
echo “I am an animal.”;
}
public function eat($food) {
echo “I am eating ” . $food . “.”;
}
}
class Dog extends Animal {
public function speak() {
echo “Woof!”;
}
public function eat($food, $quantity) {
echo “I am eating ” . $quantity . ” ” . $food . “.”;
}
}
$animal = new Animal();
$animal->speak(); // Output: I am an animal.
$animal->eat(“grass”); // Output: I am eating grass.
$dog = new Dog();
$dog->speak(); // Output: Woof!
$dog->eat(“bones”, 3); // Output: I am eating 3 bones.
In this example, we define an Animal class with two methods, speak() and eat(). We then define a Dog subclass that overrides the speak() method and overloads the eat() method with an additional parameter.
When we create an object of the Animal class and call the speak() and eat() methods, the output is “I am an animal.” and “I am eating grass.” respectively.
When we create an object of the Dog class and call the speak() and eat() methods, the output is “Woof!” and “I am eating 3 bones.” respectively, because the subclass methods override and overload the parent class methods.
28. Write a code to define constructor in php.
Ans :- In PHP, constructors are special methods that are automatically called when an object is created. They are used to initialize the object’s properties and perform any other setup that is required.
Here’s an example of how to define a constructor in PHP:
class Person {
private $name;
private $age;
public function __construct($name, $age) {
$this->name = $name;
$this->age = $age;
}
public function getName() {
return $this->name;
}
public function getAge() {
return $this->age;
}
}
$person = new Person(“John”, 30);
echo $person->getName(); // Output: John
echo $person->getAge(); // Output: 30
In this example, we define a Person class with two private properties, $name and $age. We then define a constructor method using the __construct() magic method. The constructor takes two parameters, $name and $age, and assigns their values to the corresponding properties using the $this keyword.
We also define two getter methods, getName() and getAge(), which return the values of the $name and $age properties, respectively.
When we create an object of the Person class using the new keyword and pass in values for the $name and $age parameters, the constructor is automatically called and initializes the object’s properties. We can then use the getter methods to retrieve the property values and output them to the screen.
29. Write a code to define destructor in php.
Ans :- In PHP, destructors are special methods that are automatically called when an object is destroyed or goes out of scope. They are used to perform any cleanup or resource releasing that is required.
Here’s an example of how to define a destructor in PHP:
class MyClass {
public function __construct() {
echo “Constructor called\n”;
}
public function __destruct() {
echo “Destructor called\n”;
}
}
$obj = new MyClass(); // Output: Constructor called
unset($obj); // Output: Destructor called
In this example, we define a MyClass class with a constructor method and a destructor method. The constructor simply outputs a message to the screen, while the destructor outputs a different message.
When we create an object of the MyClass class using the new keyword, the constructor is automatically called and outputs the “Constructor called” message.
When we then use the unset() function to destroy the object and release its memory, the destructor is automatically called and outputs the “Destructor called” message.
Note that in PHP, you generally do not need to define a destructor explicitly, as PHP’s garbage collector automatically cleans up objects that are no longer being used. However, if you do need to perform some specific cleanup or resource releasing when an object is destroyed, you can define a destructor as shown above.
30.Where abstract class method or function and variable implements.
Ans :- Abstract classes in PHP are used as a blueprint for other classes to extend and implement. An abstract class can contain abstract methods (i.e., methods without an implementation) and non-abstract (concrete) methods. Abstract classes cannot be instantiated, which means that you cannot create an object of an abstract class directly. Instead, you must create a subclass that extends the abstract class and implement its abstract methods.
When a class extends an abstract class that has abstract methods, it must implement those methods, which means that it must provide an implementation for each abstract method in the subclass. This is done using the abstract keyword for the method definition and the implements keyword for the class definition.
Here’s an example of an abstract class with an abstract method and a concrete method:
abstract class Animal {
protected $name;
public function __construct($name) {
$this->name = $name;
}
abstract public function makeSound();
public function getName() {
return $this->name;
}
}
class Cat extends Animal {
public function makeSound() {
echo “Meow\n”;
}
}
$cat = new Cat(“Whiskers”);
echo $cat->getName(); // Output: Whiskers
$cat->makeSound(); // Output: Meow
In this example, we define an abstract Animal class with a constructor method, an abstract makeSound() method, and a concrete getName() method.
We then define a Cat class that extends the Animal class and implements its makeSound() method by providing an implementation that outputs “Meow”.
When we create an object of the Cat class and call its getName() and makeSound() methods, we get the expected output of “Whiskers” and “Meow”, respectively.
Note that in this example, we use the abstract keyword to define the makeSound() method in the Animal class as an abstract method, and we use the implements keyword to indicate that the Cat class is implementing this method. The getName() method, on the other hand, is a concrete method that has an implementation in the Animal class, so it does not need to be implemented in the Cat class.
31.Write syntax to established connection of mysql database in php.
Ans :- To establish a connection to a MySQL database in PHP, you can use the mysqli_connect() function or the PDO class. Here’s an example of how to use mysqli_connect() to establish a connection:
// MySQL server configuration
$host = “localhost”;
$username = “root”;
$password = “password”;
$dbname = “mydatabase”;
// Connect to the database
$conn = mysqli_connect($host, $username, $password, $dbname);
// Check the connection
if (!$conn) {
die(“Connection failed: ” . mysqli_connect_error());
}
echo “Connected successfully”;
In this example, we define the MySQL server configuration variables (i.e., the host name, username, password, and database name), and then we call the mysqli_connect() function to establish a connection to the database.
If the connection is successful, the function returns a connection object that we can use to interact with the database. If the connection fails, the function returns false.
We then check the connection using an if statement and the mysqli_connect_error() function, which returns the error message if the connection fails.
If the connection is successful, we output a message to the screen saying “Connected successfully”.
Note that you should always use a secure method of storing database credentials, such as using environment variables or a configuration file that is not publicly accessible.
32. When query () and mysqli_query() used to execute query in php.
Ans :- In PHP, there are two functions that can be used to execute SQL queries: query() and mysqli_query().
The query() function is part of the MySQL extension and is used to send a SQL query to the MySQL database. However, this function has been deprecated as of PHP 5.5.0 and removed in PHP 7.0.0.
The mysqli_query() function is part of the MySQLi extension (i.e., MySQL improved) and is used to execute a SQL query on a MySQL database using a connection opened with the mysqli_connect() function.
Here’s an example of how to use mysqli_query() to execute a SQL query in PHP:
// Connect to the database
$conn = mysqli_connect(“localhost”, “username”, “password”, “mydatabase”);
// Check the connection
if (!$conn) {
die(“Connection failed: ” . mysqli_connect_error());
}
// Execute a query
$sql = “SELECT * FROM mytable”;
$result = mysqli_query($conn, $sql);
// Check if the query was successful
if ($result) {
// Output the results
while ($row = mysqli_fetch_assoc($result)) {
echo “Name: ” . $row[“name”] . ” – Age: ” . $row[“age”] . “<br>”;
}
} else {
echo “Error executing query: ” . mysqli_error($conn);
}
// Close the connection
mysqli_close($conn);
In this example, we first establish a connection to the MySQL database using mysqli_connect() and check if the connection was successful.
We then execute a SQL query using mysqli_query(), passing in the connection object and the SQL query as arguments. The function returns a result object, which we can use to fetch the results of the query.
We then check if the query was successful using an if statement, and if it was, we loop through the results using mysqli_fetch_assoc() and output each row to the screen.
If the query was not successful, we output an error message using mysqli_error().
Finally, we close the connection using mysqli_close().
33. List out any five storage engine in mysql.
Ans :- MySQL supports several storage engines, each with its own strengths and weaknesses. Here are five of the most common storage engines in MySQL, along with their syntax and a brief example:
1. MyISAM:
CREATE TABLE mytable (
id INT(11) NOT NULL AUTO_INCREMENT,
name VARCHAR(255) NOT NULL,
age INT(11) NOT NULL,
PRIMARY KEY (id)
) ENGINE=MyISAM;
MyISAM is the default storage engine for MySQL prior to version 5.5. It supports full-text searching and is best suited for read-heavy workloads. However, it does not support transactions or foreign keys.
2. InnoDB:
CREATE TABLE mytable (
id INT(11) NOT NULL AUTO_INCREMENT,
name VARCHAR(255) NOT NULL,
age INT(11) NOT NULL,
PRIMARY KEY (id)
) ENGINE=InnoDB;
InnoDB is the default storage engine for MySQL starting from version 5.5. It supports transactions and foreign keys, making it suitable for write-heavy workloads. However, it can be slower for read-heavy workloads compared to MyISAM.
3.MEMORY:
CREATE TABLE mytable (
id INT(11) NOT NULL AUTO_INCREMENT,
name VARCHAR(255) NOT NULL,
age INT(11) NOT NULL,
PRIMARY KEY (id)
) ENGINE=MEMORY;
MEMORY, also known as HEAP, is a storage engine that stores data in memory rather than on disk. This makes it very fast, but also means that the data is lost when the server is restarted. It is best suited for temporary tables or for caching frequently accessed data.
4.CSV:
CREATE TABLE mytable (
id INT(11) NOT NULL AUTO_INCREMENT,
name VARCHAR(255) NOT NULL,
age INT(11) NOT NULL,
PRIMARY KEY (id)
) ENGINE=CSV;
CSV is a storage engine that stores data in comma-separated values (CSV) format, making it easy to import and export data. However, it does not support indexes or transactions, and can be slower for complex queries.
5.BLACKHOLE:
CREATE TABLE mytable (
id INT(11) NOT NULL AUTO_INCREMENT,
name VARCHAR(255) NOT NULL,
age INT(11) NOT NULL,
PRIMARY KEY (id)
) ENGINE=BLACKHOLE;
BLACKHOLE is a storage engine that discards all data that is written to it. It is often used in replication setups where data needs to be replicated to multiple servers, but only a subset of the data needs to be stored on each server.
34.State your answer $objectname ->message() or $objectname.message() in php.
Ans :- In PHP, $objectname->message() is the syntax to call a method named message() on an object referenced by the variable $objectname.
On the other hand, $objectname.message() is not a valid syntax in PHP. It may be confused with the dot notation used in other programming languages, but in PHP the arrow -> operator is used instead to access properties or methods of an object.
35. State that php is technology,platform or programming language.
Ans :- PHP (Hypertext Preprocessor) is a server-side scripting language and a programming language, designed primarily for web development. It is often used in combination with HTML, CSS, and JavaScript to create dynamic and interactive web pages and web applications. PHP can run on various platforms and operating systems, including Windows, Linux, macOS, and others. Therefore, PHP can be considered as both a programming language and a technology.
36.Default time of session in php.
Ans :- The default time of a session in PHP is 24 minutes, which is defined by the session.gc_maxlifetime setting in the php.ini configuration file. This means that if a user is inactive for more than 24 minutes, their session data will be cleared by the garbage collector process. However, the session timeout can be changed by modifying the session.gc_maxlifetime setting or by using the session_set_cookie_params() function in PHP.
37.Write syntax to create constant variable in php.
Ans :- In PHP, a constant is a value that cannot be changed during the execution of a script. It is a named identifier that represents a specific value, like a number or a string, and can be used throughout the script.
To create a constant in PHP, you can use the define() function with the following syntax:
define(name, value, case-insensitive)
where:
name: the name of the constant, which must follow the same rules as variable names in PHP.
value: the value assigned to the constant.
case-insensitive: an optional boolean parameter that specifies whether the constant name should be case-insensitive or not. By default, it is set to false.
Here’s an example of how to define a constant in PHP:
// define a constant
define(“PI”, 3.14);
// use the constant in the script
echo “The value of PI is ” . PI;
In this example, the constant PI is defined with a value of 3.14 using the define() function. The constant is then used in the script using the echo statement to display its value.
38. What is include () function in php.
Ans :- The include() function in PHP is used to include and evaluate a specified file in the current PHP script. It is commonly used to include reusable code files or libraries in a PHP script to avoid duplicating code and to make the code more modular and maintainable.
The include() function takes a single parameter, which is the path to the file to be included. The path can be either absolute or relative to the current file, and can be a URL or a local file path. If the file specified by the path is not found or cannot be accessed, an error message is displayed and the script execution is halted.
Here’s an example of how to use the include() function in PHP:
// include a file with reusable code
include(“myfunctions.php”);
// call a function defined in the included file
$result = myfunction(3, 5);
// use the result in the script
echo “The result is: ” . $result;
In this example, the include() function is used to include a file called myfunctions.php, which contains some reusable code. The file is then evaluated and any functions or variables defined in it become available in the current script. A function called myfunction() is called with two arguments, and the result is stored in a variable called $result. Finally, the result is displayed in the output using the echo statement.
39.What is header() function in php.
Ans :- The header() function in PHP is used to send a raw HTTP header to the client browser. It allows you to send additional information, such as content type, caching instructions, redirection instructions, and more, along with the response data.
The header() function takes a single parameter, which is the string of the header to be sent. The header string must conform to the HTTP specification and include both the header name and its value. Multiple headers can be sent in a single script execution by calling the header() function multiple times with different headers.
Here’s an example of how to use the header() function in PHP:
// set the content type to plain text
header(‘Content-Type: text/plain’);
// set a custom header
header(‘X-Custom-Header: Hello World!’);
// redirect the user to another page
header(‘Location: http://www.example.com/’);
In this example, the header() function is used to set the content type to plain text, send a custom header called X-Custom-Header with the value of Hello World!, and redirect the user to another page. Note that the header(‘Location: …’) function is used to trigger a browser redirection to the specified URL. When sending a Location header, no other output should be sent before the header, and the script should exit immediately after sending the header to avoid any further processing.
40. What is used of move_uploaded_file() in php.
Ans :- The move_uploaded_file() function in PHP is used to move a file that was uploaded via a form to a new location on the server. When a file is uploaded using PHP’s $_FILES array, it is temporarily stored in a temporary directory on the server. The move_uploaded_file() function is used to move this file from the temporary directory to a permanent location on the server.
The move_uploaded_file() function takes two parameters: the temporary filename of the uploaded file, and the destination filename to which the file should be moved. The function returns true if the file was successfully moved, and false otherwise.
Here’s an example of how to use the move_uploaded_file() function in PHP:
<?php
// get the temporary filename of the uploaded file
$temp_filename = $_FILES[‘file’][‘tmp_name’];
// create the destination filename
$destination_filename = ‘/path/to/uploads/’ . $_FILES[‘file’][‘name’];
// move the file from the temporary directory to the uploads directory
if (move_uploaded_file($temp_filename, $destination_filename)) {
echo ‘File uploaded successfully.’;
} else {
echo ‘Error uploading file.’;
}
?>
In this example, the move_uploaded_file() function is used to move the uploaded file to a new location on the server. The temporary filename of the uploaded file is obtained from the $_FILES array, and the destination filename is created by concatenating the desired upload directory with the original filename of the uploaded file. If the file is moved successfully, the script outputs a success message; otherwise, it outputs an error message.
Answer the following question.
1.Explain Feature of php.
Ans :-PHP (Hypertext Preprocessor) is a server-side scripting language widely used for web development. Here are some key features of PHP:
Easy to learn: PHP syntax is similar to other programming languages such as C and Java, making it easy to learn for those with programming experience.
Platform independent: PHP code runs on various platforms including Windows, Linux, and macOS, which means it can be deployed on almost any web server.
Open source: PHP is an open-source language, which means it is freely available for anyone to download and use.
Dynamic and flexible: PHP is a dynamic language that can be used for a variety of purposes, including web development, command-line scripting, and desktop application development.
Server-side scripting: PHP code runs on the server, which means that it can be used to create dynamic web pages that respond to user input.
Extensive library support: PHP has a large number of libraries that can be used to quickly build complex web applications.
Database support: PHP has built-in support for many popular databases, including MySQL, PostgreSQL, and Oracle.
Object-oriented programming (OOP): PHP supports OOP, allowing developers to write more modular and reusable code.
Security: PHP has many built-in security features, such as input validation and data filtering, which help to prevent common security vulnerabilities.
Scalability: PHP can handle high traffic websites with ease and can be scaled up to handle even the largest websites.
2. Explain data types in php.
Ans :- PHP (Hypertext Preprocessor) is a dynamically typed language, which means that the data type of a variable is determined automatically based on the value assigned to it. However, there are certain data types in PHP that are used to represent specific types of data. Here are the main data types in PHP:
String: A string is a sequence of characters, enclosed in single or double quotes. Strings are used to represent textual data.
Integer: An integer is a whole number without a decimal point. Integers are used to represent numeric data.
Float (or Double): A float is a number with a decimal point. Floats are used to represent decimal or fractional values.
Boolean: A Boolean value can be either true or false. Booleans are used to represent logical values.
Array: An array is a collection of values, each identified by a unique key. Arrays are used to store and manipulate multiple values.
Object: An object is an instance of a class. Objects are used to represent complex data structures.
Null: Null is a special data type that represents a variable with no value.
Resource: A resource is a special variable that holds a reference to an external resource, such as a database connection or a file handle.
In addition to these basic data types, PHP also supports more advanced data types such as classes, interfaces, and traits that are used in object-oriented programming.
3. What is php.ini file and how php code in parsed.
Ans :- The php.ini file is a configuration file used by PHP to customize the behavior of the PHP interpreter. It contains settings that affect the way PHP operates, such as the maximum file upload size, the error reporting level, and the maximum memory usage.
The php.ini file is typically located in the PHP installation directory or in the web server’s configuration directory. It can be edited using a text editor to change the values of the various settings.
When a PHP script is executed, the PHP code is parsed and executed by the PHP interpreter. Here’s a brief overview of how this process works:
The web server receives a request for a PHP script.
The PHP interpreter is invoked to parse the script.
The PHP interpreter reads the script line by line and executes it.
Any output generated by the script is sent back to the web server, which then sends it back to the client’s browser.
During the parsing process, the PHP interpreter looks for PHP code enclosed in opening and closing tags (<?php and ?>). The interpreter then executes the PHP code and generates output based on the instructions in the code. The output can be HTML, text, images, or any other type of data.
In summary, the php.ini file is used to configure the behavior of the PHP interpreter, while the PHP code is parsed and executed by the interpreter to generate dynamic content for the web server.
4.How to create array in php.explain types of array with suitable example.
Ans :- In PHP, an array is a data structure that stores one or more values of the same data type. You can create an array in PHP using the array() function. Here’s an example:
$fruits = array(“apple”, “banana”, “orange”);
This creates an array called $fruits with three elements: “apple”, “banana”, and “orange”.
PHP supports several types of arrays, including:
1. Numeric Arrays: A numeric array stores a sequence of values that are accessed using numeric indices. Here’s an example:
$numbers = array(1, 2, 3, 4, 5);
2. Associative Arrays: An associative array stores key-value pairs, where each key is a string and each value can be of any data type. Here’s an example
$person = array(
“name” => “John Doe”,
“age” => 30,
“email” => “john.doe@example.com”
);
3.Multidimensional Arrays: A multidimensional array stores arrays as its elements. These arrays can be either numeric or associative arrays. Here’s an example:
$students = array(
array(“name” => “John”, “age” => 20, “email” => “john@example.com”),
array(“name” => “Jane”, “age” => 21, “email” => “jane@example.com”),
array(“name” => “Bob”, “age” => 22, “email” => “bob@example.com”)
);
5.How to create foreach loop in php and when it is used in php.
Ans:- In PHP, a foreach loop is used to iterate over the elements of an array or an object. The basic syntax of a foreach loop in PHP is as follows:
foreach ($array as $value) {
// Code to be executed for each element of the array
}
Here, $array is the array that you want to iterate over, and $value is a variable that will hold the value of the current element of the array in each iteration of the loop. You can choose any variable name for $value.
Here’s an example of how to use a foreach loop to iterate over an array:
$fruits = array(“apple”, “banana”, “orange”);
foreach ($fruits as $fruit) {
echo $fruit . “<br>”;
}
In this example, the foreach loop iterates over the $fruits array and assigns each element of the array to the $fruit variable in each iteration of the loop. The echo statement then prints the value of $fruit to the screen, with a line break (<br>) at the end of each line.
The foreach loop is used when you want to iterate over the elements of an array or an object, and perform some operation on each element. For example, you might use a foreach loop to display a list of items on a webpage, or to calculate the total value of an order by iterating over the items in the order. The foreach loop is a powerful tool in PHP that can help you to efficiently process large amounts of data.
6.Explain associative array in php with suitable example.
Ans :- In PHP, an associative array is an array where each element has a unique key assigned to it. Unlike numeric arrays, which use sequential integer keys, associative arrays use string keys to access their values. You can create an associative array in PHP using the array() function, and specifying the keys and values of each element within the array. Here’s an example:
$person = array(
“name” => “John Doe”,
“age” => 30,
“email” => “john.doe@example.com”
);
In this example, $person is an associative array with three elements: “name”, “age”, and “email”. The key of each element is a string, and the value of each element can be of any data type.
To access the value of a specific element in an associative array, you can use its key as an index. Here’s an example:
echo $person[“name”];
This will output “John Doe” to the screen.
You can also use a foreach loop to iterate over the elements of an associative array. Here’s an example:
foreach ($person as $key => $value) {
echo $key . “: ” . $value . “<br>”;
}
In this example, the foreach loop iterates over the $person array and assigns each key-value pair to the $key and $value variables in each iteration of the loop. The echo statement then prints the key and value of each element to the screen, separated by a colon and space (: ), with a line break (<br>) at the end of each line.
The associative array is a powerful tool in PHP that allows you to store and access data in a structured and flexible way. You can use associative arrays to represent complex data structures, such as objects or database records, or to map values to specific keys for easy access and retrieval.
7.Explain sorting function in array with suitable example.
Ans :- In PHP, you can use sorting functions to sort the elements of an array in a specific order. PHP provides several built-in sorting functions, including sort(), rsort(), asort(), arsort(), ksort(), and krsort(). Here’s an explanation of each of these functions, with suitable examples:
1. sort(): This function sorts the elements of an array in ascending order, based on their values. Here’s an example:
$numbers = array(4, 2, 8, 5, 1);
sort($numbers);
print_r($numbers);
This will output:
Array
(
[0] => 1
[1] => 2
[2] => 4
[3] => 5
[4] => 8
)
2.rsort(): This function sorts the elements of an array in descending order, based on their values. Here’s an example:
$numbers = array(4, 2, 8, 5, 1);
rsort($numbers);
print_r($numbers);
This will output:
Array
(
[0] => 8
[1] => 5
[2] => 4
[3] => 2
[4] => 1
)
3.asort(): This function sorts the elements of an array in ascending order, based on their values, while maintaining the key-value associations. Here’s an example
$person = array(
“name” => “John Doe”,
“age” => 30,
“email” => “john.doe@example.com”
);
asort($person);
print_r($person);
This will output:
Array
(
[age] => 30
[email] => john.doe@example.com
[name] => John Doe
)
4.arsort(): This function sorts the elements of an array in descending order, based on their values, while maintaining the key-value associations. Here’s an example:
$person = array(
“name” => “John Doe”,
“age” => 30,
“email” => “john.doe@example.com”
);
arsort($person);
print_r($person);
This will output:
Array
(
[name] => John Doe
[email] => john.doe@example.com
[age] => 30
)
5.ksort(): This function sorts the elements of an array in ascending order, based on their keys. Here’s an example:
$person = array(
“name” => “John Doe”,
“age” => 30,
“email” => “john.doe@example.com”
);
ksort($person);
print_r($person);
This will output:
Array
(
[age] => 30
[email] => john.doe@example.com
[name] => John Doe
)
6.krsort(): This function sorts the elements of an array in descending order, based on their keys. Here’s an example:
$person = array(
“name” => “John Doe”,
“age” => 30,
“email” => “john.doe@example.com”
);
krsort($person);
print_r($person);
This will output:
Array
(
[name] => John Doe
[email] =>
8.Explain$_GET,$_POST,$_SESSION,$_COOKIE in php.
Ans :- In PHP, $_GET, $_POST, $_SESSION, and $_COOKIE are superglobal variables used to store data across requests. Here’s an explanation of each of these variables:
1. $_GET: This variable is used to retrieve data sent in the URL query string. This is often used to pass data between web pages. Here’s an example:
http://example.com/page.php?name=John&age=30
In this example, the values of name and age can be retrieved using the $_GET variable like this:
$name = $_GET[‘name’];
$age = $_GET[‘age’];
2. $_POST: This variable is used to retrieve data sent in the HTTP request body. This is often used for submitting forms or sending data that should not be visible in the URL. Here’s an example:
<form method=”post” action=”process.php”>
<input type=”text” name=”username”>
<input type=”password” name=”password”>
<input type=”submit” value=”Submit”>
</form>
In this example, the values of username and password can be retrieved using the $_POST variable in the process.php script like this:
$username = $_POST[‘username’];
$password = $_POST[‘password’];
3. $_SESSION: This variable is used to store data across multiple requests for a specific user. This is often used for maintaining user authentication and user-specific settings. Here’s an example:
session_start();
$_SESSION[‘username’] = ‘JohnDoe’;
In this example, the value of username is stored in the $_SESSION variable and can be accessed in subsequent requests like this:
session_start();
$username = $_SESSION[‘username’];
4. $_COOKIE: This variable is used to store data on the client-side, often for storing user preferences or tracking user behavior. Here’s an example:
setcookie(‘username’, ‘JohnDoe’, time() + 3600);
In this example, the value of username is stored in a cookie that will expire in one hour. The value of the cookie can be retrieved in subsequent requests like this:
$username = $_COOKIE[‘username’];
9.Explain Session in php with suitable example.
Ans :-In PHP, a session is a way to store data across multiple requests for a specific user. This is often used for maintaining user authentication and user-specific settings.
Here’s an example of how to use sessions in PHP:
1. Starting a Session
To start a session, you need to call the session_start() function at the beginning of your PHP script. Here’s an example:
session_start();
2.Setting Session Variables
Once the session has started, you can set session variables using the $_SESSION superglobal variable. Here’s an example:
$_SESSION[‘username’] = ‘JohnDoe’;
3.Accessing Session Variables
To access the value of a session variable, you simply need to reference it using the $_SESSION superglobal variable. Here’s an example:
$username = $_SESSION[‘username’];
4.Destroying a Session
To destroy a session and all of its associated data, you can call the session_destroy() function. Here’s an example
session_destroy();
In this example, the session is destroyed and all of the data associated with it is removed.
Here’s a complete example that demonstrates how to use sessions in PHP:
<?php
session_start();
// Set session variables
$_SESSION[‘username’] = ‘JohnDoe’;
$_SESSION[’email’] = ‘johndoe@example.com’;
// Access session variables
$username = $_SESSION[‘username’];
$email = $_SESSION[’email’];
echo “Username: $username<br>”;
echo “Email: $email<br>”;
// Destroy the session
session_destroy();
?>
In this example, we start a session, set two session variables, access the session variables, and then destroy the session.
10. Explain Cookie in php with suitable example.
Ans :- In PHP, a cookie is a small piece of data that is stored on the client-side, often for storing user preferences or tracking user behavior.
Here’s an example of how to use cookies in PHP:
1. Setting a Cookie
To set a cookie, you can use the setcookie() function. Here’s an example:
setcookie(‘username’, ‘JohnDoe’, time() + 3600);
In this example, we’re setting a cookie called username with a value of JohnDoe that will expire in one hour (specified by time() + 3600).
2. Accessing a Cookie
To access the value of a cookie, you can use the $_COOKIE superglobal variable. Here’s an example:
$username = $_COOKIE[‘username’];
In this example, we’re retrieving the value of the username cookie and storing it in the $username variable.
3.Updating a Cookie
To update a cookie, you can simply set it again using the setcookie() function. Here’s an example:
setcookie(‘username’, ‘JaneDoe’, time() + 3600);
In this example, we’re updating the value of the username cookie to JaneDoe
4. Deleting a Cookie
To delete a cookie, you can set it with an expiration time in the past. Here’s an example:
setcookie(‘username’, ”, time() – 3600);
In this example, we’re setting the value of the username cookie to an empty string and setting the expiration time to one hour ago, effectively deleting the cookie.
Here’s a complete example that demonstrates how to use cookies in PHP:
<?php
// Set a cookie
setcookie(‘username’, ‘JohnDoe’, time() + 3600);
// Access the cookie
$username = $_COOKIE[‘username’];
echo “Username: $username<br>”;
// Update the cookie
setcookie(‘username’, ‘JaneDoe’, time() + 3600);
// Delete the cookie
setcookie(‘username’, ”, time() – 3600);
?>
In this example, we set a cookie called username with a value of JohnDoe, accessed the value of the cookie, updated the value of the cookie to JaneDoe, and then deleted the cookie.
11.Explain any five file handling function in php.
Ans :-In PHP, file handling functions are used to read, write, and manipulate files. Here are five commonly used file handling functions:
fopen(): This function is used to open a file in PHP. It takes two parameters: the file name (including the path) and the mode in which the file should be opened. The mode can be read-only (‘r’), write-only (‘w’), or read-write (‘r+’ or ‘w+’), among others.
fgets(): This function is used to read a single line from an open file. It takes one parameter: the file handle returned by fopen(). It returns the next line of the file, or false if the end of the file has been reached.
fwrite(): This function is used to write to an open file. It takes two parameters: the file handle returned by fopen(), and the string to be written to the file. It returns the number of bytes written, or false if an error occurred.
fclose(): This function is used to close an open file. It takes one parameter: the file handle returned by fopen(). After a file has been closed, no further operations can be performed on it until it is opened again.
file_exists(): This function is used to check if a file exists on the server. It takes one parameter: the file name (including the path). It returns true if the file exists, and false otherwise.
Here’s an example that demonstrates how to use these functions to read and write to a file in PHP:
<?php
// Open the file in write mode
$file = fopen(‘example.txt’, ‘w’);
// Write a string to the file
fwrite($file, ‘This is a test.’);
// Close the file
fclose($file);
// Open the file in read mode
$file = fopen(‘example.txt’, ‘r’);
// Read a line from the file
$line = fgets($file);
// Output the line
echo $line;
// Close the file
fclose($file);
// Check if the file exists
if (file_exists(‘example.txt’)) {
echo ‘The file exists.’;
} else {
echo ‘The file does not exist.’;
}
?>
In this example, we first open a file in write mode, write a string to the file using fwrite(), and then close the file using fclose(). We then open the same file in read mode, read a line from the file using fgets(), output the line, and then close the file again using fclose(). Finally, we check if the file exists using file_exists() and output a message accordingly.
12.Explain String ,Math function in php.
Ans :- String functions in PHP:
strlen(): This function is used to find the length of a string.
str_replace(): This function is used to replace a part of a string with another string.
strpos(): This function is used to find the position of the first occurrence of a substring within a string.
strtolower(): This function is used to convert a string to lowercase.
strtoupper(): This function is used to convert a string to uppercase.
substr(): This function is used to extract a substring from a string.
Here’s an example that demonstrates how to use some of these string functions in PHP:
<?php
$string = “Hello, World!”;
// Find the length of the string
echo strlen($string); // Output: 13
// Replace ‘World’ with ‘PHP’
echo str_replace(‘World’, ‘PHP’, $string); // Output: Hello, PHP!
// Find the position of ‘World’ in the string
echo strpos($string, ‘World’); // Output: 7
// Convert the string to lowercase
echo strtolower($string); // Output: hello, world!
// Convert the string to uppercase
echo strtoupper($string); // Output: HELLO, WORLD!
// Extract the substring ‘World’ from the string
echo substr($string, 7, 5); // Output: World
?>
Math functions in PHP:
abs(): This function is used to find the absolute value of a number.
sqrt(): This function is used to find the square root of a number.
pow(): This function is used to find the power of a number.
max(): This function is used to find the maximum value of two or more numbers.
min(): This function is used to find the minimum value of two or more numbers.
Here’s an example that demonstrates how to use some of these math functions in PHP:
<?php
$num1 = 10;
$num2 = -5;
// Find the absolute value of num2
echo abs($num2); // Output: 5
// Find the square root of num1
echo sqrt($num1); // Output: 3.1622776601684
// Find the power of num1 to the 3rd
echo pow($num1, 3); // Output: 1000
// Find the maximum value of num1 and num2
echo max($num1, $num2); // Output: 10
// Find the minimum value of num1 and num2
echo min($num1, $num2); // Output: -5
?>
13.Explain different storage engine in php.
Ans :- In PHP, the term “storage engine” typically refers to the different ways in which data can be stored in a database system. The most commonly used storage engines in PHP are:
MyISAM: This is the default storage engine in MySQL prior to version 5.5. It is a non-transactional storage engine that is known for its speed and simplicity. It is best suited for read-intensive applications, such as websites that have a lot of traffic.
InnoDB: This is the default storage engine in MySQL from version 5.5 onwards. It is a transactional storage engine that supports features such as row-level locking, foreign key constraints, and crash recovery. It is best suited for applications that require high concurrency and transactional support.
Memory: This is a storage engine that allows data to be stored in memory instead of on disk. It is best suited for applications that require fast access to frequently accessed data, such as caching.
CSV: This is a storage engine that allows data to be stored in comma-separated value (CSV) format. It is best suited for applications that require data to be imported or exported in CSV format.
Blackhole: This is a storage engine that discards all data that is written to it. It is primarily used for testing or for applications that need to discard data quickly.
NDB: This is a storage engine that is used with the MySQL Cluster database. It is a distributed storage engine that provides high availability and scalability. It is best suited for applications that require high availability and real-time performance.
Each storage engine has its own strengths and weaknesses, and the choice of storage engine depends on the specific needs of the application. For example, if the application requires transactional support, InnoDB is the best choice, while if the application requires fast access to frequently accessed data, the memory engine is the best choice.
14.Explain object oriented concept in php.
Ans :- Object-oriented programming (OOP) is a programming paradigm that focuses on the use of objects to represent and manipulate data. In PHP, object-oriented programming is implemented through classes and objects.
A class is a blueprint or template for creating objects. It defines the properties and methods that an object of that class will have. A class can be thought of as a user-defined data type, with its own set of attributes and behavior.
An object is an instance of a class. It is created using the “new” keyword followed by the name of the class. Once an object is created, its properties and methods can be accessed using the object operator (->).
Here is an example of a simple class in PHP:
class Person {
// properties
public $name;
public $age;
// methods
public function sayHello() {
echo “Hello, my name is ” . $this->name . ” and I am ” . $this->age . ” years old.”;
}
}
In this example, the Person class has two properties, $name and $age, and one method, sayHello(). The sayHello() method uses the $name and $age properties to output a greeting.
To create an object of the Person class, we use the following code:
$person = new Person();
We can then set the values of the $name and $age properties using the object operator:
$person->name = “John”;
$person->age = 30;
Finally, we can call the sayHello() method using the object operator:
$person->sayHello(); // outputs “Hello, my name is John and I am 30 years old.”
This is a very basic example, but it demonstrates the fundamentals of object-oriented programming in PHP. By creating classes and objects, we can encapsulate data and behavior in a modular and reusable way. This makes our code easier to maintain, and allows us to build complex applications with relative ease.
15.Write script to connect mysql database in php also write script for create table ,insert, update delete, view records.
Ans :- PHP script that demonstrates how to connect to a MySQL database, create a table, insert data, update data, delete data, and view records:
<?php
// Database connection settings
$servername = “localhost”;
$username = “yourusername”;
$password = “yourpassword”;
$dbname = “yourdatabase”;
// Create connection
$conn = new mysqli($servername, $username, $password, $dbname);
// Check connection
if ($conn->connect_error) {
die(“Connection failed: ” . $conn->connect_error);
}
// Create table
$sql = “CREATE TABLE MyGuests (
id INT(6) UNSIGNED AUTO_INCREMENT PRIMARY KEY,
firstname VARCHAR(30) NOT NULL,
lastname VARCHAR(30) NOT NULL,
email VARCHAR(50),
reg_date TIMESTAMP DEFAULT CURRENT_TIMESTAMP ON UPDATE CURRENT_TIMESTAMP
)”;
if ($conn->query($sql) === TRUE) {
echo “Table MyGuests created successfully”;
} else {
echo “Error creating table: ” . $conn->error;
}
// Insert data
$sql = “INSERT INTO MyGuests (firstname, lastname, email)
VALUES (‘John’, ‘Doe’, ‘john@example.com’)”;
if ($conn->query($sql) === TRUE) {
echo “New record created successfully”;
} else {
echo “Error: ” . $sql . “<br>” . $conn->error;
}
// Update data
$sql = “UPDATE MyGuests SET lastname=’Doe Jr.’ WHERE id=1”;
if ($conn->query($sql) === TRUE) {
echo “Record updated successfully”;
} else {
echo “Error updating record: ” . $conn->error;
}
// Delete data
$sql = “DELETE FROM MyGuests WHERE id=1”;
if ($conn->query($sql) === TRUE) {
echo “Record deleted successfully”;
} else {
echo “Error deleting record: ” . $conn->error;
}
// View records
$sql = “SELECT id, firstname, lastname, email FROM MyGuests”;
$result = $conn->query($sql);
if ($result->num_rows > 0) {
while($row = $result->fetch_assoc()) {
echo “id: ” . $row[“id”]. ” – Name: ” . $row[“firstname”]. ” ” . $row[“lastname”]. ” – Email: ” . $row[“email”]. “<br>”;
}
} else {
echo “0 results”;
}
$conn->close();
?>
Note that you will need to replace the $servername, $username, $password, and $dbname variables with your own database connection details, and modify the SQL statements to suit your specific needs.
16.Explain inheritance in php with suitable example
Ans :- Inheritance is a key feature of object-oriented programming that allows you to define a new class based on an existing class, thereby inheriting its properties and methods. In PHP, you can achieve inheritance using the extends keyword.
Here’s an example to demonstrate inheritance in PHP:
class Animal {
public $name;
public $species;
function __construct($name, $species) {
$this->name = $name;
$this->species = $species;
}
function eat() {
echo “{$this->name} is eating.”;
}
}
class Cat extends Animal {
function meow() {
echo “{$this->name} is meowing.”;
}
}
// Creating a new instance of the Cat class
$myCat = new Cat(“Whiskers”, “Feline”);
// Accessing properties and methods from the parent Animal class
echo $myCat->species; // Outputs “Feline”
$myCat->eat(); // Outputs “Whiskers is eating.”
// Accessing methods from the child Cat class
$myCat->meow(); // Outputs “Whiskers is meowing.”
In the example above, we first define an Animal class with two properties and a method. We then define a Cat class that extends the Animal class using the extends keyword. The Cat class adds its own method, meow(), to the inherited properties and methods of the Animal class.
We then create a new instance of the Cat class and use it to access properties and methods from both the parent Animal class and the child Cat class. This demonstrates how inheritance allows you to reuse code and build more specialized classes on top of existing ones.




Popular Videos

UX for Teams
Learn the basics and a bit beyond to improve your backend dev skills.

Designer

SEO & Instagram
Learn the basics and a bit beyond to improve your backend dev skills.

Designer